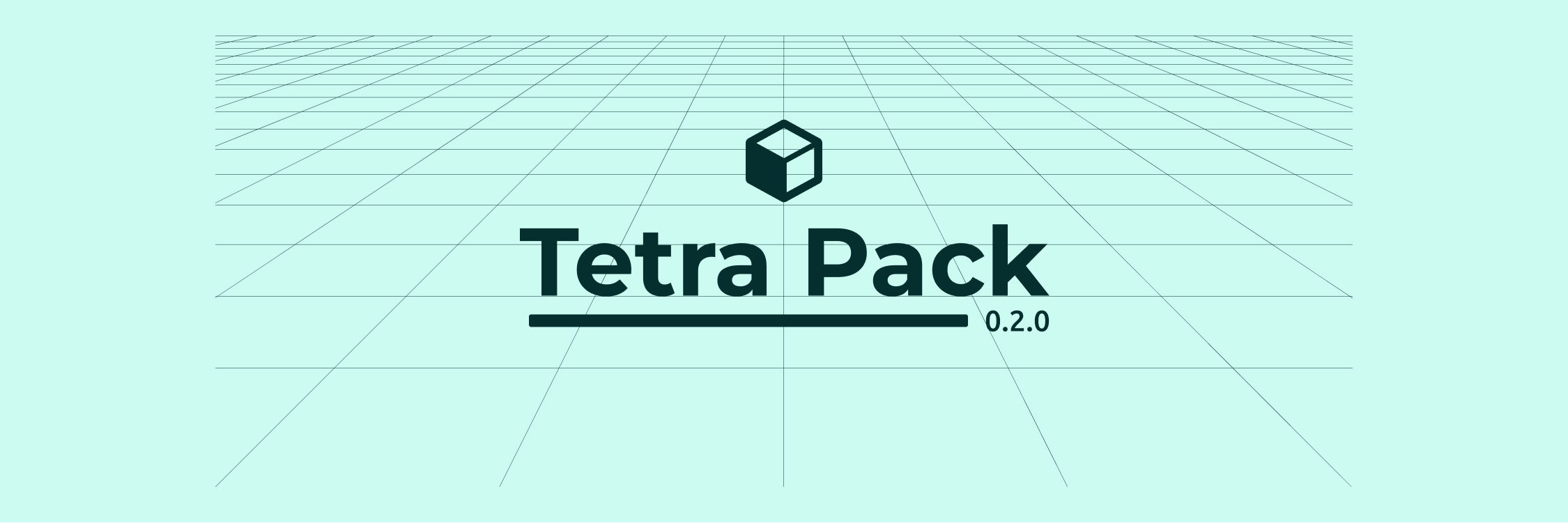
Tetra Pack
A simple and configurable notion API parser for react projects.
Features -
- Robust and granular Parser
- Keyed Approach
- Configurable blocks and annotations
- Loose Type Checking
Remember
- It isn't bundled with
@notion/client
. Hence, you need to require it externally. - It renders pre-cooked API data into pure html tags.
- It follows keyed approach.
Every element is rendered with a unique key to avoid UI mis-match or clashes.
- All the blocks are configurable and can be replaced or extended.
Installation
Bootstrapped Installation
The best way to get started is to clone the NEXT.JS and tailwind CSS starter template (opens in a new tab) from Github and follow the steps given in README.
git clone https://github.com/ashishk1331/tetra-example.git
Manual Installation
To add Tetra-Pack to an existing project, simply install it via npm
npm install tetrapack
:::tip Remember It does not concern TetraPack how you obtain your notion data. You can use any pattern or technique to acquire the same. ::: Here, we'll show one of the ways to get Notion page data and render it using TetraPack.
-
First install notion client (opens in a new tab) as the basic dependency
npm i @notionhq/client
-
Get your integration token from notion developers (opens in a new tab) dashboard.
-
Copy the Page ID of the notion page you want to render, from the share option at the top right corner. PAGE ID = https://www.notion.so/Tetra-Pack-401aa30875db4f97b2d4e698b328ad45?pvs=4 (opens in a new tab) This is your id = 401aa30875db4f97b2d4e698b328ad45
-
Now create a .env file at the root level, having all details.
NOTION_TOKEN = <NOTION_INTEGRATION_TOKEN> NOTION_PAGE_ID = <NOTION_PAGE_ID>
-
Create a function to fetch page data
async function getBlocks(pageId) { const notion = new Client({ auth: process.env.NOTION_TOKEN, }); let data = await notion.blocks.children.list({ block_id: pageId, page_size: 50, }); return data.results; }
-
Now pass the blocks array, returned from the fetch function, as the prop to the Parser.
<Parser blocks={blocks} />
And voila you've rendered whole page using TetraPack!